Android Pingを使ってデバイスの接続を検索
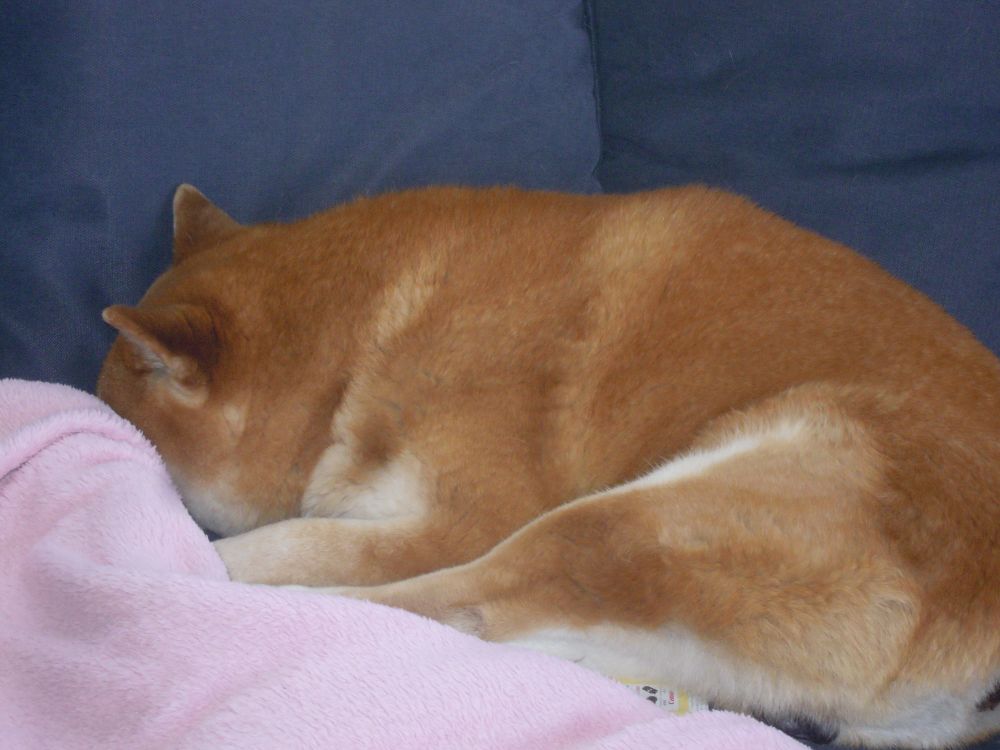
デバイスの接続検索に、そんなに時間がかかるのか!おれはもう寝るといって寝ている柴犬です。
概要
前回、Socket でローカルネットワークに接続しているデバイスの検索を試みましたが、時間がかなり要するので別の方法を考えることにしました。
考えた方法が Ping を使ってみることでした。
実行させてみたところ、これもかなりの時間を要する結果となりました。
これを記録することにします。
翔泳社 齊藤 新三 著
2024年2月26日現在
WEBのみでは断片的で覚えにくいので最初に購入した Kotlin の本です。
Ping の処理
Ping の処理は次のブロックを subnet、ubnet2 の for ループで繰り返しています。
String ipaddress = "192.168." + subnet2 + "." + subnet;
String tempcmd = "ping -c 5 " + ipaddress;
Log.d("startPing","開始 :" + ipaddress);
Runtime runtime = Runtime.getRuntime();
Process proc = null;
try {
proc = runtime.exec(tempcmd);
proc.waitFor();
} catch (Exception e) {
sl.add("err ip: " + ipaddress);
}
int exitVal = proc.exitValue();
if (exitVal == 0) {
sl.add("ip: " + ipaddress + "-->PING OK");
Log.d("startPing","PING :" + ipaddress + " OK");
} else {
sl.add("ip: " + ipaddress + "-->PING NG");
Log.d("startPing","PING :" + ipaddress + " NG");
}
MainActivity.java
package org.sibainu.relax.room.myapplication; import java.net.InetSocketAddress; import java.net.Socket; import java.util.ArrayList; import android.os.AsyncTask; import android.os.Bundle; import android.util.Log; import android.view.View; import android.widget.Button; import android.widget.TextView; import androidx.appcompat.app.AppCompatActivity; public class MainActivity extends AppCompatActivity { @Override protected void onCreate(Bundle savedInstanceState) { super.onCreate(savedInstanceState); setContentView(R.layout.activity_main); ClickListener cl = new ClickListener(); Button btn; btn = findViewById(R.id.button); btn.setOnClickListener(cl); btn = findViewById(R.id.button2); btn.setOnClickListener(cl); } // ---------- private class ClickListener implements View.OnClickListener { @Override public void onClick(View view) { int num = view.getId(); if (num == R.id.button) { Log.d("startScan","開始"); startScan(); } else if (num == R.id.button2) { Log.d("startPing","開始"); startPing(); } } } // ---------- private void startScan() { ----------------略---------------- } // ---------- private void startPing() { try { TextView tv; tv = findViewById(R.id.textView2); // タスクの実行 new PingStateTask() { @Override protected void onPostExecute(String response) { if (response == "1") { Log.d("NetworkStateTask","success"); } } }.execute(tv); } catch (Exception e){ Log.d("Exception", "err: " + e); } } // ---------- private class NetworkStateTask extends AsyncTask<TextView, Integer, String> { ----------------略---------------- } // ---------- private class PingStateTask extends AsyncTask<TextView, Integer, String> { @Override protected String doInBackground(TextView... params) { ArrayList<String> sl = new ArrayList<>(); for (int subnet2 = 0; subnet2 < 2; subnet2++) { for (int subnet = 0; subnet < 256; subnet++) { String ipaddress = "192.168." + subnet2 + "." + subnet; String tempcmd = "ping -c 5 " + ipaddress; Log.d("startPing","開始 :" + ipaddress); Runtime runtime = Runtime.getRuntime(); Process proc = null; try { proc = runtime.exec(tempcmd); proc.waitFor(); } catch (Exception e) { sl.add("err ip: " + ipaddress); } int exitVal = proc.exitValue(); if (exitVal == 0) { sl.add("ip: " + ipaddress + "-->PING OK"); Log.d("startPing","PING :" + ipaddress + " OK"); } else { sl.add("ip: " + ipaddress + "-->PING NG"); Log.d("startPing","PING :" + ipaddress + " NG"); } } } String response = ""; if (sl.size() > 0) { response = String.join("\n",sl); } params[0].setText(response); return "1"; } } }
実行結果
ひとつのアドレスに5秒以上かかっていますので、192.168.0.X が終わるのに5秒✕256=1280秒以上要することになります。
これも時間がかかるようです。
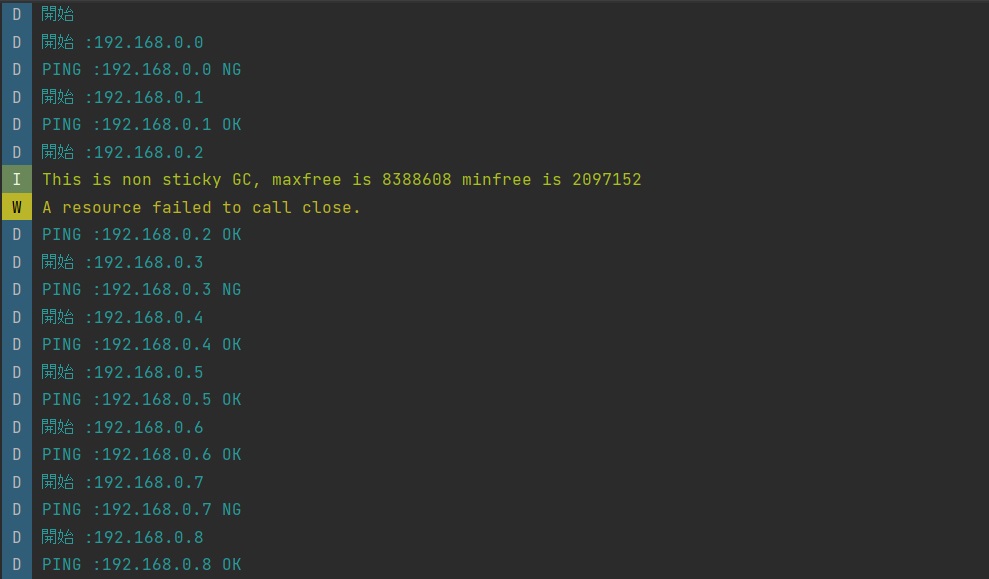
192.168.1.X になったら、ひとつのアドレスに14秒以上かかるようになりました。
原因はわかりません。終わるのに1時間以上要しますのでここで止めます。
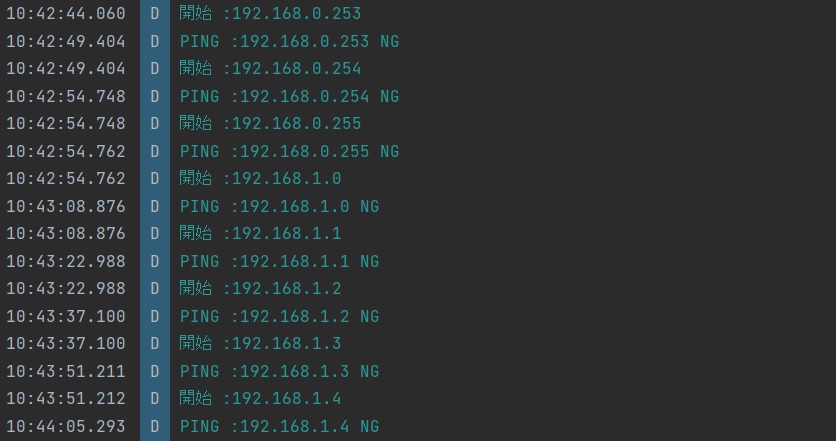
activity_main.xml
画面のレイアウトは、ボタンと TextView がそれぞれ2つ配置しています。
<?xml version="1.0" encoding="utf-8"?> <androidx.constraintlayout.widget.ConstraintLayout xmlns:android="http://schemas.android.com/apk/res/android" xmlns:app="http://schemas.android.com/apk/res-auto" xmlns:tools="http://schemas.android.com/tools" android:layout_width="match_parent" android:layout_height="match_parent" tools:context=".MainActivity"> <Button android:id="@+id/button" android:layout_width="wrap_content" android:layout_height="wrap_content" android:layout_marginTop="24dp" android:text="Port" app:layout_constraintEnd_toEndOf="parent" app:layout_constraintStart_toStartOf="parent" app:layout_constraintTop_toTopOf="parent" /> <TextView android:id="@+id/textView" android:layout_width="0dp" android:layout_height="225dp" android:layout_marginTop="8dp" android:text="TextView" app:layout_constraintEnd_toEndOf="parent" app:layout_constraintHorizontal_bias="0.0" app:layout_constraintStart_toStartOf="parent" app:layout_constraintTop_toBottomOf="@+id/button" /> <Button android:id="@+id/button2" android:layout_width="wrap_content" android:layout_height="wrap_content" android:layout_marginTop="32dp" android:text="Ping" app:layout_constraintEnd_toEndOf="parent" app:layout_constraintStart_toStartOf="parent" app:layout_constraintTop_toBottomOf="@+id/textView" /> <TextView android:id="@+id/textView2" android:layout_width="0dp" android:layout_height="0dp" android:layout_marginTop="8dp" android:layout_marginBottom="16dp" android:text="TextView" app:layout_constraintBottom_toBottomOf="parent" app:layout_constraintEnd_toEndOf="parent" app:layout_constraintHorizontal_bias="1.0" app:layout_constraintStart_toStartOf="parent" app:layout_constraintTop_toBottomOf="@+id/button2"/> </androidx.constraintlayout.widget.ConstraintLayout>
タブレットの表示
放置して気が付いたら終わっていました。
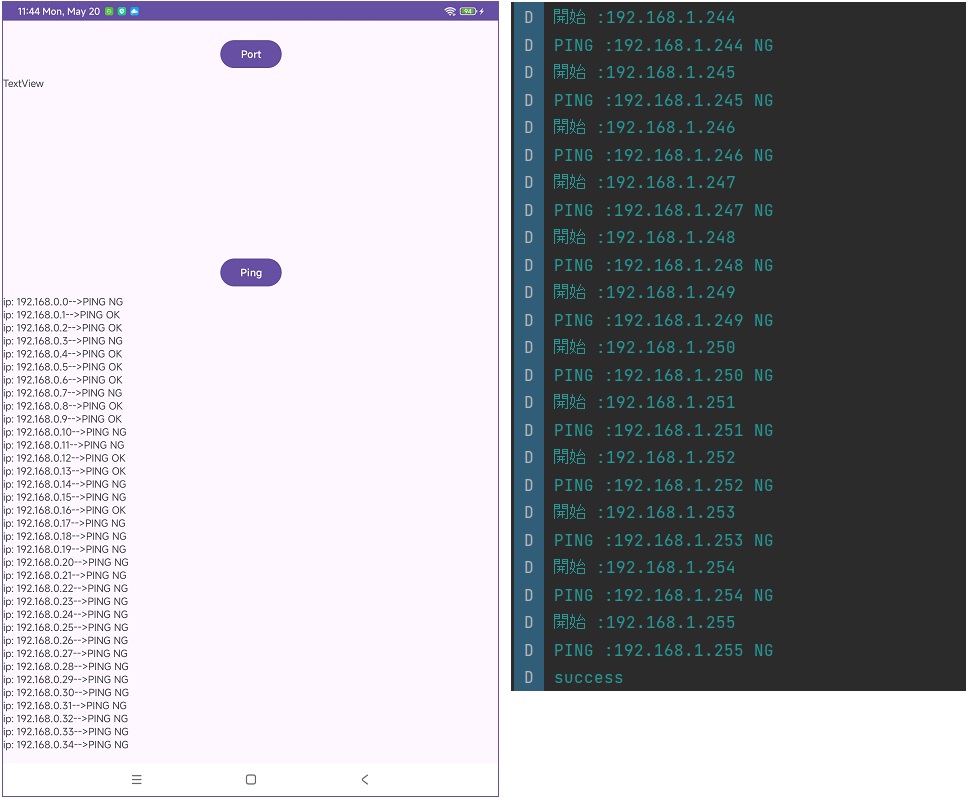
ここまでとします。