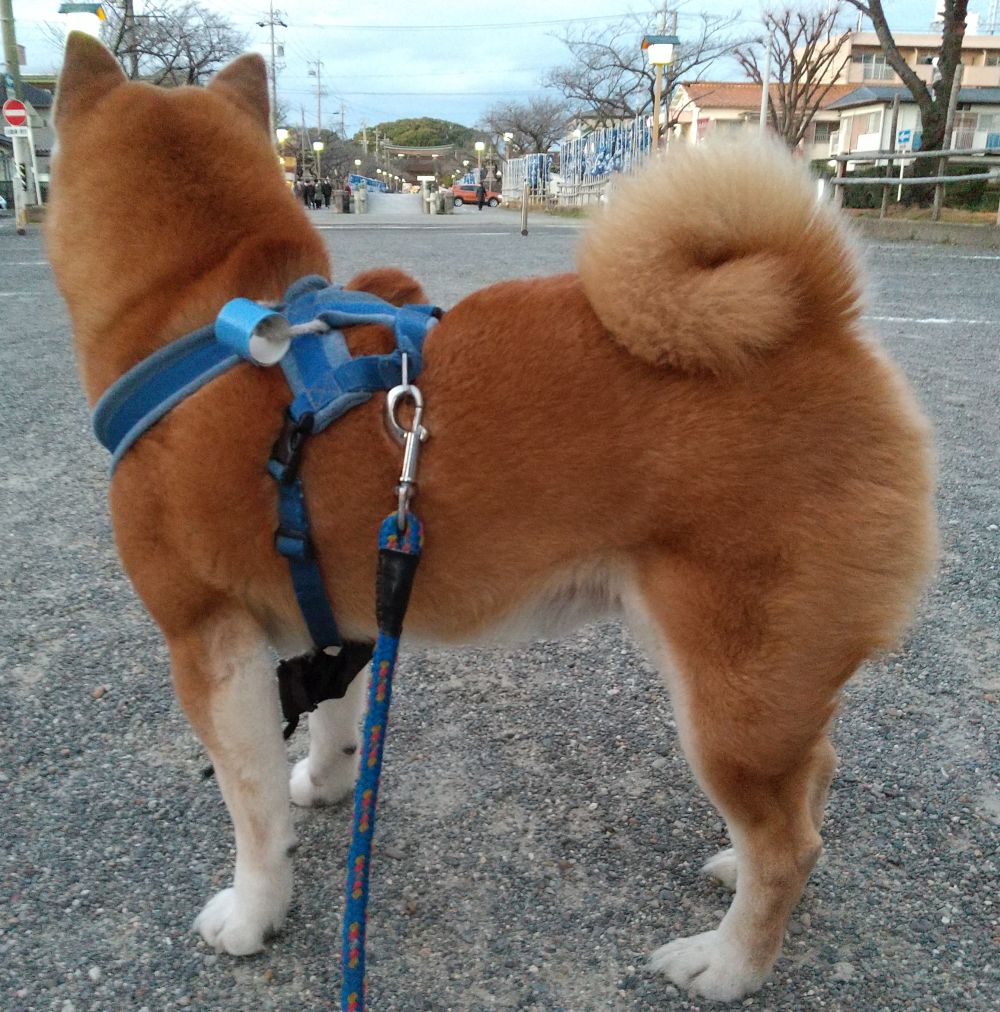
お正月の騒がしさも収まって静かになったなあと思う柴犬です。
概要
Python で遊んでいます。
複数のリストからタプルを作る必要があり、調べました。
せっかくですから、その方法を記録することにしました。
map関数を使います。
ところで、Python は勿論、RUST にも map 関数があり iterable を最適に扱う上で必要不可欠となっているようです。
map 関数
Pythonの公式ページのドキュメントには次のように書かれています。
これが一番よく理解できました。
https://docs.python.org/3/library/functions.html?highlight=map#map
map(function, iterable, *iterables)
Return an iterator that applies function to every item of iterable, yielding the results.
If additional iterables arguments are passed, function must take that many arguments and is applied to the items from all iterables in parallel.
With multiple iterables, the iterator stops when the shortest iterable is exhausted.
要約すると、次のように解釈しました。
(内部の)関数[function]をイテレータの動作をするすべての事項に適用してその結果を集積したイテレータを返します。
2つ目以降にイテレータの動作をする引数があれば、(内部の)関数はその引数に作用し並行してすべてのその引数の項目に適用します。
イテレータの動作をするもの(引数)が複数の場合、一番短いイテレータの動作をするものが吐き出されたらイテレータは終了します。
例
4タプルをキーを付加して1つのタプル
次のタプルのリストにたいして
iter1 = [("a11","a12"),("a21","a22"),("a31","a32")]
iter2 = [("b11","b12"),("b21","b22"),("b31","b32")]
iter3 = [("c11","c12"),("c21","c22"),("c31","c32")]
iter4 = [("d11","d12"),("d21","d22"),("d31","d32")]
キーを iter1の2つ目にします。次の map 関数を適用すると
list(map(lambda a, b, c, d: (a[1], a, b, c, d), iter1, iter2, iter3, iter4))
次のようなリストが返されます。
[('a12', ('a11', 'a12'), ('b11', 'b12'), ('c11', 'c12'), ('d11', 'd12')),
('a22', ('a21', 'a22'), ('b21', 'b22'), ('c21', 'c22'), ('d21', 'd22')),
('a32', ('a31', 'a32'), ('b31', 'b32'), ('c31', 'c32'), ('d31', 'd32'))]
ですので、2行目を取得したいならば、次のような関数にします。
list(map(lambda a, b, c, d: (a[1], a, b, c, d), iter1, iter2, iter3, iter4))[1]
返されるタプルは
('a22', ('a21', 'a22'), ('b21', 'b22'), ('c21', 'c22'), ('d21', 'd22'))
となります。
列並びを変える
また、2列3タプルを3列2タプルにすることもできます。次のようにすると
iter1 = [(1,2),(10,20),(1000,2000)]
iter2 = [(100,200),(110,210),(1100,2100)]
iter3 = [(10,20),(20,30),(2000,3000)]
list(map(lambda a, b, c: ((a[0] + 10, a[1] + 10, b[0] + 50), (b[1] + 60, c[0] + 100, c[1] + 500)), iter1, iter2, iter3))
このように3列2タプルが返されます。
[((11, 12, 150), (260, 110, 520)),
((20, 30, 160), (270, 120, 530)),
((1010, 2010, 1150), (2160, 2100, 3500))]
タプルだけではない
iterable なものならタプルに限りません。
例えば、次のようなリストを考えます。
最初と同じことをしてみます。
iter1 = [["a11","a12"],["a21","a22"],["a31","a32"]]
iter2 = [["b11","b12"],["b21","b22"],["b31","b32"]]
iter3 = [["c11","c12"],["c21","c22"],["c31","c32"]]
iter4 = [["d11","d12"],["d21","d22"],["d31","d32"]]
list(map(lambda a, b, c, d: [a[1], a, b, c, d], iter1, iter2, iter3, iter4))
すると次のようなリストが返されます。
[['a12', ['a11', 'a12'], ['b11', 'b12'], ['c11', 'c12'], ['d11', 'd12']],
['a22', ['a21', 'a22'], ['b21', 'b22'], ['c21', 'c22'], ['d21', 'd22']],
['a32', ['a31', 'a32'], ['b31', 'b32'], ['c31', 'c32'], ['d31', 'd32']]]
非常に活用範囲が広いものとなっています。